多层神经网络的Tensorflow实战
- 神经元的Tensorflow实现
- 神经网络的Tensorflow实现
Tensorflow环境搭建
1 2 3 4
| conda install tensorflow==1.8.0 下载数据集CIFAR-10 python version conda install jupyter jupyter notebook
|
数据预处理
加载数据集
1 2 3 4 5 6
| import pickle import numpy as np import os
CIFAR_DIR = 'cifar-10-batches-py' print(os.listdir(CIFAR_DIR))
|
[‘batches.meta’, ‘test_batch’, ‘data_batch_1’, ‘data_batch_4’, ‘data_batch_5’, ‘readme.html’, ‘data_batch_2’, ‘data_batch_3’]
查看数据类型
1 2 3 4 5 6 7 8 9 10
| with open(os.path.join(CIFAR_DIR,"data_batch_1"),'rb') as f: data = pickle.load(f,encoding='bytes') print(type(data)) print(data.keys()) print(type(data[b'data']),type(data[b'labels']),type(data[b'batch_label']),type(data[b'filenames'])) print(data[b'data'].shape)#3072 = r-g-b = 1024 * 3 print(data[b'data'][0:2]) print(data[b'labels'][0:2]) print(data[b'batch_label']) print(data[b'filenames'][0:2])
|
<class ‘dict’>
dict_keys([b’batch_label’, b’labels’, b’data’, b’filenames’])
<class ‘numpy.ndarray’> <class ‘list’> <class ‘bytes’> <class ‘list’>
(10000, 3072)
[[ 59 43 50 … 140 84 72]
[154 126 105 … 139 142 144]]
[6, 9]
b’training batch 1 of 5’
[b’leptodactylus_pentadactylus_s_000004.png’, b’camion_s_000148.png’]
验证图片输出
1 2 3 4 5 6 7 8 9
| image_arr = data[b'data'][100] image_arr = image_arr.reshape((3,32,32))# 32 32 3 image_arr = image_arr.transpose((1,2,0))
import matplotlib.pyplot as plt from matplotlib.pyplot import imshow %matplotlib inline
imshow(image_arr)
|
<matplotlib.image.AxesImage at 0x7f5f48c41898>
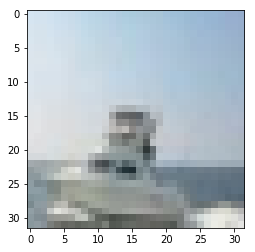